Mastering JavaScript URL Encoding for Your Web Applications
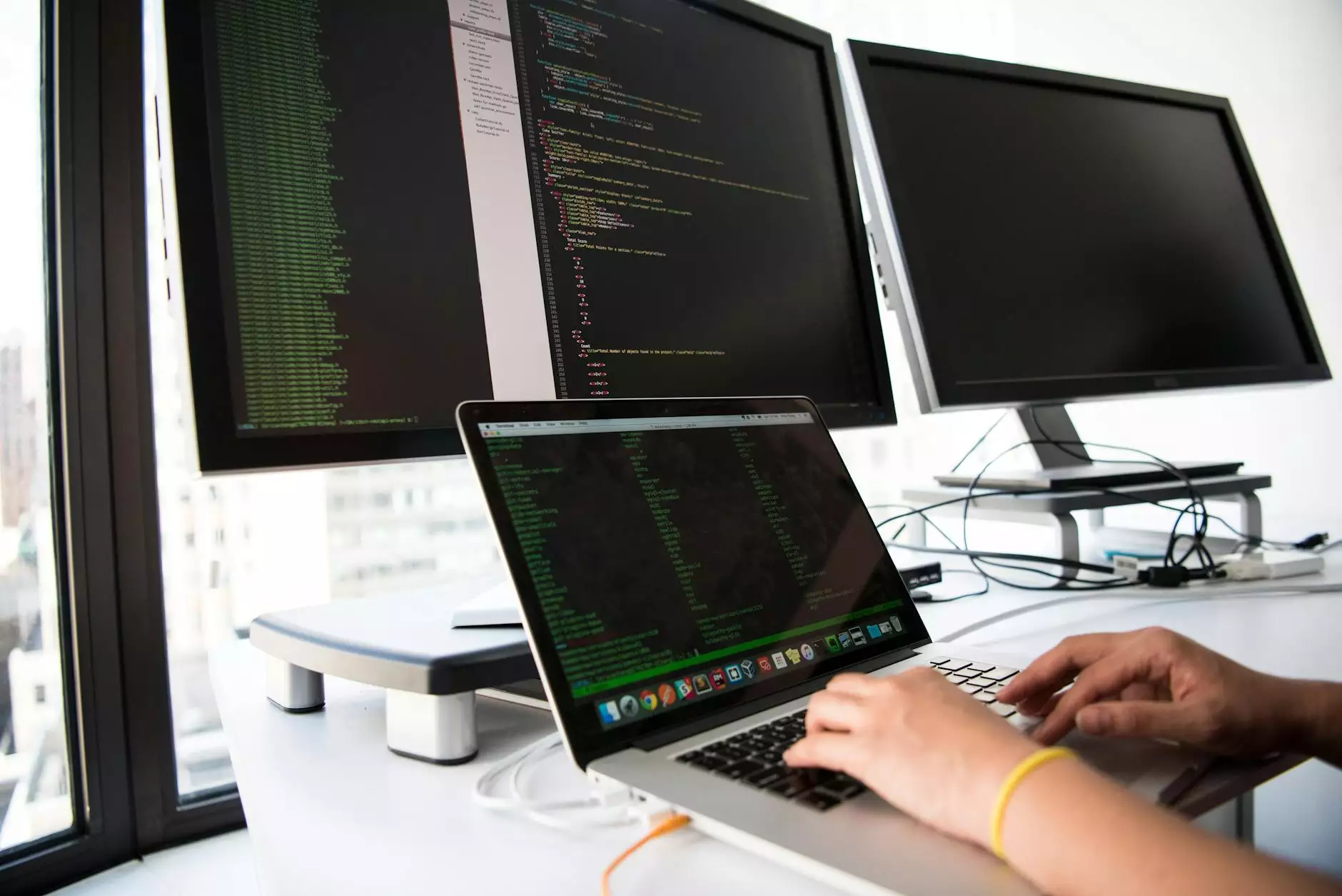
JavaScript URL encoding is an essential skill for developers and web designers alike. In today's digital landscape, understanding how to properly encode URLs can significantly impact your application's functionality and security. In this comprehensive guide, we will delve deep into the intricacies of URL encoding, explore its importance, and provide practical examples to enhance your web projects. Whether you're designing a new website or developing software solutions, mastering this topic can lead you to remarkable results.
What is URL Encoding?
URL encoding, also known as percent encoding, is a way to convert characters into a format that can be transmitted over the Internet. URLs can only be sent over the Internet using the ASCII character set, which contains 128 symbols. This means that characters outside this set, such as Unicode characters, must be converted to a format that can be handled more effectively. URL encoding replaces unsafe ASCII characters with a "%" followed by two hexadecimal digits, ensuring that the URL is valid and can be properly interpreted by web servers and browsers.
Why is URL Encoding Important?
Understanding the importance of URL encoding is crucial for several reasons:
- Security: URL encoding prevents various injections and attacks, such as Cross-Site Scripting (XSS) and SQL Injection, by ensuring that special characters are correctly interpreted.
- Data Integrity: By encoding data, you can ensure that it maintains its integrity when transferred over different systems, avoiding corruption or loss of information.
- Compatibility: Different systems and software may interpret special characters differently. URL encoding creates a standard format that ensures compatibility across various platforms.
- User Experience: Properly encoded URLs can improve user experience by ensuring that links redirect users to the correct pages, even when special characters are present.
How to Encode URLs in JavaScript
In JavaScript, encoding URLs is straightforward with built-in functions. The primary functions used for URL encoding are encodeURIComponent() and encodeURI(). Each function serves a unique purpose, so it's crucial to understand their differences.
1. Using encodeURIComponent()
The encodeURIComponent() function is designed to encode individual components of a URI. This function is particularly useful when you want to encode query string parameters or URI fragments. For example:
const param = "hello world!"; const encodedParam = encodeURIComponent(param); console.log(encodedParam); // Output: hello%20world%21In this example, the space character is replaced with %20, and the exclamation mark is replaced with %21, making the string safe to include in a URL.
2. Using encodeURI()
On the other hand, the encodeURI() function is used to encode complete URIs. It does not encode characters that have special meanings in a URI, such as the colon (:), forward slash (/), and question mark (?). This is useful when you want to encode a full URL without altering its structural components. For example:
const url = "https://www.example.com/search?q=hello world!"; const encodedURL = encodeURI(url); console.log(encodedURL); // Output: https://www.example.com/search?q=hello%20world!In this case, only the space is encoded, while other characters remain unchanged.
Common Use Cases for URL Encoding
URL encoding plays a critical role in various scenarios, including:
1. Submitting Form Data
When forms on a website are submitted, the data is sent through URLs. Proper encoding of user inputs is essential to prevent issues with data processing and ensure that the server can interpret the information correctly. For instance:
const userInput = "John Doe & Associates"; const safeInput = encodeURIComponent(userInput); // Use safeInput in your form submission2. Creating Dynamic Links
Dynamic web applications often require generating URLs that include user-generated data. Encoding these strings is vital to prevent broken links or incorrect redirections:
function createLink(baseURL, userID) { return `${baseURL}/profile?id=${encodeURIComponent(userID)}`; }3. Ensuring API Request Validity
When interacting with APIs, it's common to include parameters in the request URL. Ensuring these parameters are properly encoded can prevent errors and guarantee the request is processed:
const apiUrl = "https://api.example.com/data?query=" + encodeURIComponent("JavaScript URL Encode");Understanding URL Decoding
While encoding is crucial, understanding URL decoding is equally important. URL decoding is the process of converting percent-encoded strings back into their original format. In JavaScript, this is accomplished using the decodeURIComponent() and decodeURI() functions.
1. Using decodeURIComponent()
The decodeURIComponent() function is used to decode individual components of a URI:
const encodedParam = "hello%20world%21"; const decodedParam = decodeURIComponent(encodedParam); console.log(decodedParam); // Output: hello world!2. Using decodeURI()
The decodeURI() function is for decoding complete URIs. It preserves special characters:
const encodedURL = "https://www.example.com/search?q=hello%20world%21"; const decodedURL = decodeURI(encodedURL); console.log(decodedURL); // Output: https://www.example.com/search?q=hello world!Best Practices for URL Encoding
To ensure effective URL encoding, consider the following best practices:
- Always Encode User Inputs: Any data received from users should be encoded before using it in URLs to prevent potential security vulnerabilities.
- Use encodeURIComponent() for Parameters: When encoding components, always prefer to use encodeURIComponent() for parameter values.
- Decode When Necessary: Always decode strings when retrieving data from URLs to present it in a readable format.
- Test URLs: After encoding or decoding URLs, always test them to ensure they function as expected.
Conclusion
In conclusion, mastering JavaScript URL encoding is fundamental for web developers and designers. By understanding how to properly encode and decode URLs, you enhance the security, compatibility, and functionality of your web applications. Remember to use the right functions for the right scenarios, and always prioritize the safety of your applications by encoding user inputs. By applying these principles, you are well on your way to becoming a proficient developer capable of creating secure and effective web solutions at semalt.tools.
Further Reading
If you're interested in expanding your knowledge on web design and software development, consider exploring the following topics:
- Web Design Fundamentals
- Advanced Software Development Techniques
- Comprehensive JavaScript Tutorials